AI Agent for Email Automation with SmolAgents
A practical guide to building an email agent using Hugging Face's smolagents, Slack notifications, and calendar integrations
About the author: Louis-François Bouchard is a co-founder and CTO of Towards AI, a leading platform for AI education that has taught over 400,000 users since 2019. With a background in biomedical engineering and AI research, he brings a unique perspective to his work. Through his YouTube channel 'What's AI' and his newsletter, he shares insights into the latest AI developments and their implications.
There has been a lot of confusion about AI agents lately and even experts sometimes find themselves juggling with multiple definitions. What people call an AI agent is often “just” an AI workflow—a distinction that is crucial to make. It determines whether a system operates with rigid predictability or dynamic adaptability, which directly impacts how tasks are handled, the complexity of the system, and operational costs.
The terms ‘workflows’ and ‘agent’ are widely used interchangeably, adding confusion to an already complex landscape. Anthropic, in their recent article building effective agents, clarified this distinction by clearly defining both. It defines workflows as:
Workflows are systems where LLMs and tools are orchestrated through predefined code paths.
On the other hand,
Agents are systems where LLMs dynamically direct their own processes and tool usage, maintaining control over how they accomplish tasks.
OpenAI, at The AI Engineer Summit 2025 NYC, defined agents as following:
An Al application consisting of a model equipped with instructions that guide its behavior, access to tools that extend its capabilities, encapsulated in a runtime with a dynamic lifecycle.
The key distinction here is “dynamic lifecycle”, which distinguishes an agent from a workflow. Where given a task, and a set of tools, the agent itself orchestrates the execution path instead of taking a pre-defined path with some variation.
In this article, we will demonstrate how to build an email agent using the smolagents
library by HuggingFace. This agent will have access to essential tools, including a calendar, the ability to send Slack notifications for important emails, and the capability to process incoming emails and generate responses when needed. Additionally, we will discuss when to use agents and when to avoid them, and best practices for their implementation.
Editor’s note: Further exploring this distinction, our guest author clearly differentiates workflows from agents in one of his YouTube videos, pointing out that:
Workflows are pretty predictable and consistent, focusing on structured tasks performed consistently. In contrast, true agents are capable of functioning independently, employing processes akin to human reasoning, reflecting flexibility and autonomy.
When to Use and Avoid Agents
Given the interchangeability of agents and workflows, it's important to understand when to use the dynamic capabilities of agents versus sticking with simpler workflows. Below are some basic principles to help determine whether agents are necessary for your workflows.
When to Use AI Agents
AI agents are useful in scenarios where decision-making depends on variable factors and needs flexibility; it is hard to pre-define an execution path. Unlike traditional rule-based systems that adhere to fixed patterns, agents can process diverse inputs and adjust their decision-making flow in real-time.
Devin is a great example of an agent that functions like a software engineer, striving to accomplish a coding task. It operates dynamically with tools at its disposal, such as browsing the internet for documentation, executing code, and adapting its actions based on the results, to complete its task. The steps taken during such a task have a degree of autonomy and are difficult to define in advance.
In contrast, a workflow in this case operates like a meticulous project manager, following a predefined sequence, consulting specific documentation, executing code, and verifying outcomes. Each action is predetermined, ensuring consistency and predictability. The process is linear and structured, with each step clearly defined beforehand.
While this agent’s flexible nature enables us to automate tasks that would otherwise not have been possible, it also introduces a higher level of non-determinism, as it’s not possible to foresee every action. It takes careful planning to develop a domain-specific agent that can successfully achieve a particular task.
When to Avoid AI Agents
More often than not workflows are a better fit for many AI systems. In processes where inputs and outputs follow a predictable pattern, such as a RAG pipeline, text manipulation, or document processing pipeline, a system with a pre-defined flow is more efficient and effective with a deterministic nature. Introducing an agent in these scenarios can add unnecessary complexity when dynamic decision-making or variable inputs handling is not required.
Consider a document processing pipeline as an example. A workflow-based system could use an LLM to extract key information, classify documents, and generate summaries based on predefined rules. In a legal setting, specialized AI platforms like Harvey AI can simplify tasks such as reviewing documents, spotting problematic clauses, and recommending changes to ensure compliance. By using fine-tuned models trained on legal data, Harvey AI delivers precise insights, making analysis faster and more efficient. This structured approach ensures consistency and reliability using LLMs’ strengths in language understanding without requiring the adaptability of an agent.
In general, it's good practice to take the time to break down your problem into its fundamental components before moving forward. If you would like to practice this, we cover the whole stack step by step in our From Beginner to Advanced LLM Developer course. By identifying the key elements, you can simplify requirements and more easily determine what your system truly needs. This approach will also help you select the right tools to develop your AI agent or workflow.
Now, let's dive into the details of designing AI agents.
Building Agents in Production: Email Agent
💡 You can access the complete colab notebook with the code for this article here.
Here we have chosen a fairly simple agent to help you connect the dots between theory and real-world application. This practical implementation will help you explore the fundamental concepts of AI agents and understand how agents dynamically interact with tools, make decisions, and adapt to different scenarios.
You can always make iterative improvements to increase autonomy, customization, etc. as your understanding of the fundamentals grows.
Our approach is mostly inspired by what OpenAI recommended in their aforementioned talk.
To begin, start with small, focused applications. Avoid unnecessary frameworks and understand the fundamental AI components. This comes in handy when you later scale your system for complex use cases so you know where to go in and optimize.
Incremental autonomy. While AI agents are good at autonomy, defining clear input, output, and action parameters ensures they remain reliable and aligned with requirements. For critical actions, human-in-loop is a good practice.
Use guardrails to maintain safety while handling edge cases effectively. This helps keep your system secure. For example, lightweight guardrails for an email agent would validate email content before processing. In practice, use an LLM to validate the email content.
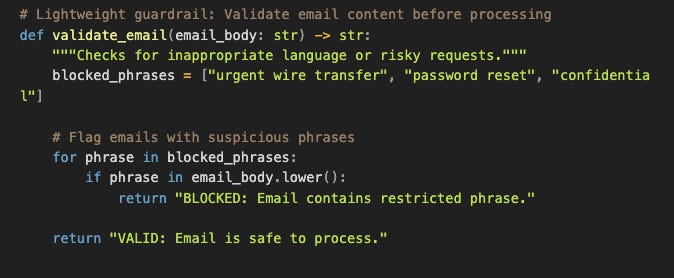
Plan for failures. Like any system, AI agents will encounter unexpected scenarios. Implementing fallback mechanisms, such as human escalation for unresolved queries, improves user experience.
Now, let’s create a simple email agent to help us better understand how agents work and interact.
In this example, we will build an email agent by giving it access to our calendar, web, and Slack. The goal of building such an agent is to automate business emails, where given an email, it can look up our calendar for availability, search on the web for information if needed, and notify on Slack for any critical email along with the summary.
We will use the smolagents library by Hugging Face to build our Email Agent. It is a lightweight, open-source framework for building AI agents. It allows developers to build AI-powered agents without dealing with unnecessary complexity. More on smolagents here.
Step 1: Setup
Install
smolagents
andhuggingface_hub
packages in your Python env:
pip install smolagents huggingface_hub
huggingface_hub
is required for auth with huggingface for using LLM models.
Import the following utilities and smolagents components:
from typing import Dict, Optional
from datetime import datetime, timedelta
from smolagents import CodeAgent, HfApiModel, tool
Below are the details of the imported components:
CodeAgent
: Enables smolagents to generate and execute Python code snippets (including tool calls)HfApiModel
: Connects to Hugging Face's API to access language models.tool
: It is a@tool
decorator, used to define LLM tool calls, required to interact with external systems.
Step 2: Tool Calls
Tool 1: Calendar
It gives access to the appropriate calendar to the agent. In our case, it takes a date and duration parameter as input. It mimics a calendar system to check availability and automate scheduling. By managing meeting requests, it helps decide whether to accept, reschedule, or suggest alternatives.
For example, if an email asks, “Can we meet tomorrow at 2 PM for 90 minutes?” the tool checks availability and responds accordingly. In practice, you’d want to connect to your calendar via an API.
Tool 2: Web Search
This tool allows our agent to do a web search and return the result to LLM. This helps the agent to look up the web to get extra information and use that context to generate informed and grounded responses.
For example, if someone emails asking "What are the current API rate limits?", the agent can use this tool to search for that information and include it in the response without needing to guess or make up details.
Tool 3: Slack
Slack tool allows the agent to interact with the Slack, so it can identify you or your team of any critical email. We have defined this tool to take a dictionary with email processing details, formats it into a structured notification, and prints it to the console (simulating a Slack message).
Define Email Agent
To define our agent, we first specify the model, i.e., mistralai/Mistral-7B-Instruct-v0.2
in this case, followed by the system prompt containing its purpose, tool usage, and examples. Then we create the agent and pass all the parameters.
Currently, our implementation does not customize the agent to capture a person’s response style, but this can be added. By incorporating user preferences, such as formality, tone, verbosity, greeting style, and signature, the agent can adapt to a person's email writing style.
Once set up, it handles the rest.
Testing
We define a set of dummy email examples to simulate the email processing, and then run the agent for each. Below are the emails that we use:
Then we pass each email to our agent:
The outputs of each run look like this:
The logs show that the agent successfully processed the meeting request by checking the calendar for the requested date (2025-02-24) and time (14:00) for a 90-minute meeting. It confirmed the availability of the 14:00-16:00 slot and scheduled the meeting. The agent then created a Slack notification to inform the team about the meeting’s purpose, availability check result, scheduled time, and next steps. Finally, the agent provided a clear confirmation message that the meeting was scheduled at the preferred time.
If you want to implement your email agent, you can access and follow along the complete notebook here.
Final Thoughts
AI agents excel at dynamic decision-making by orchestrating their tool usage, while workflows follow predefined paths. Our email automation example, though guided by a structured prompt, shows how an agent can choose which tools to use, decide the order of actions, and combine information from calendars and web searches in real time. This goes beyond following static instructions by adapting to whatever the email needs, especially when email requests become ambiguous or span multiple domains.
We chose a simple example with smolagents to show how AI agents can adapt to different scenarios without jumping straight into a fully autonomous setup. While our design includes some workflow-like guidelines, the agent still has freedom to decide how it carries out each step. For straightforward or predictable tasks, a workflow might be enough and is often easier to manage. However, as email requests become more varied and complex, an agent’s autonomy, adaptability, and dynamic tool orchestration stand out. Ultimately, the key is to match your approach to your needs: use agents only when flexibility is essential; start small, implement guardrails, and plan for failures. For predictable tasks, simpler workflows often provide better efficiency and reliability.
The key to building effective AI agents is knowing when to prioritize flexibility over structure—and how to design for both. If you're ready to build ‘real’ agents for your complex workflows, organization, or startup, our From Beginner to Advanced LLM Developer course covers exactly that. You'll learn how to build AI agents that can autonomously handle complex tasks and get access to the complete LLM Developer toolkit that prepares you for the industry.
Super excited to see the article live!! I was glad to work with you on this one Pascal :)
I enjoyed the step by step simple example. It’s a great overview for beginners.
I was thinking about it though and wanted your opinion. If you were going to build this particular scenario for real you’d have at least 2 agents collaborating would you not.
The web tool would likely be handled by a “Research Agent” while the other 2 tools probably live in a single “Personal Assistant” agent.
I know you were trying to keep this a simple, “this is why and where you’d use agents” example but I thought I’d add a bit of more context here in the comments.
Of course please disagree if you feel that all 3 tools would indeed like in a single Agent - in this case a “Personal Assistant” agent.
Cheers,
Christopher